- if (Gain@3kHz - avg Hearing Threshold Level ) >= Gain@Peak - avg Hearing Threshold Level), then:
- Adjust Gain Control / Volume Control to match Soft Target at 3000Hz.
- else
- match Gain Control / Volume Control to frequency with peak gain.
- match equalizer frequencies to Soft Target from 5.2 - 7.2 kHz range up to midpoint (i.e. if frequency is matched at greater than midpoint, reset it to midpoint).
EqMax := NzBase.GetParamMax('BEQ1_gain'); TargetCurve := sdSoft; Case TargetCurve of sdSoft: TargetCurveValue := 40; sdModerate: TargetCurveValue := 60; else TargetCurveValue := 80; end; // Adjust GC/VC to match Soft target at 3000 Hz. // However, if HearingLossThreshold at 500Hz > HearingLossThreshold at 1000Hz, // and HearingLossThreshold at 1000Hz > HearingLossThreshold at 2000Hz, skip // {if (Audiogram.Values[fq500] <= Audiogram.Values[fq1000]) or // (Audiogram.Values[fq1000] <= Audiogram.Values[fq2000]) then} // Get frequency of peak gain HighestFreq := fq3000; if Audiogram.Values[HighestFreq] < Audiogram.Values[fq2000] then HighestFreq := fq2000; if Audiogram.Values[HighestFreq] < Audiogram.Values[fq1000] then HighestFreq := fq1000; if Audiogram.Values[HighestFreq] < Audiogram.Values[fq500] then HighestFreq := fq500; // Get avg HearingLossThreshold AvgThres := (Audiogram.Values[fq500] + Audiogram.Values[fq1000] + Audiogram.Values[fq2000] + Audiogram.Values[fq3000]) div 4; // compare avg HearingLossThreshold to audiogram values at 3kHz and Peak frequency if (Audiogram.Values[fq3000]-AvgThres) >= (Audiogram.Values[HighestFreq]-AvgThres) then begin // if freq3000 contains peak, we simply adjust GC/VC to match Soft Target at 3000 Hz. AdjustParam(TargetCurve, 'matrix_gain', 0, 47, 1, 47 {2.997kHz}, TargetCurveValue); end else begin // if frequencies other than freq3000 contains peak gain, // we adjust GC/VC to match Soft Target at that other frequency. Case HighestFreq of fq500 : AdjustParam(TargetCurve, 'matrix_gain', 0, 47, 1, 16 {500Hz}, TargetCurveValue); fq1000: AdjustParam(TargetCurve, 'matrix_gain', 0, 47, 1, 28 {1000Hz}, TargetCurveValue); fq2000: AdjustParam(TargetCurve, 'matrix_gain', 0, 47, 1, 40 {2000Hz}, TargetCurveValue); end; // Match equalizers frequencies to Soft Target from 5.2 - 7.2 kHz. SetParamValue('BEQ10_gain', FindBestMatch(TargetCurve, 'BEQ10_gain', 0, EqMax, 57 {5339Hz}, TargetCurveValue), False); // 5.2 kHz SetParamValue('BEQ11_gain', FindBestMatch(TargetCurve, 'BEQ11_gain', 0, EqMax, 60 {6350Hz}, TargetCurveValue), False); // 6.5 kHz SetParamValue('BEQ12_gain', FindBestMatch(TargetCurve, 'BEQ12_gain', 0, EqMax, 62 {7127Hz}, TargetCurveValue), False); // 7.2 kHz end;== Main Algorithms == Autofit routine dependencies:
- Normal (WDRC) autofit ⇒ Autofit Defaults
- Normal (WDRC) autofit ⇒ Calculate VC Range
- Normal (WDRC) autofit ⇒ Match 3kHz or appropriate peak
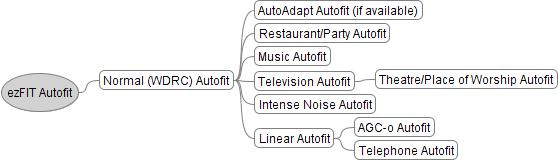
- Apply Autofit Defaults
- Turn on mute (if available)
- Adjust compression ratios in each channel based on the following rules based on the audiometric thresholds 500Hz, 1kHz, 2kHz, 3kHz, & 4kHz:
- if HearingThresholdLevel < = 35dB, then CR = 1:1
- else if HearingThresholdLevel < = 40dB, then CR = 1.11:1 (or closest value)
- else if HearingThresholdLevel < = 45dB, then CR = 1.18:1 (or closest value)
- else if HearingThresholdLevel < = 50dB, then CR = 1.33:1 (or closest value)
- else if HearingThresholdLevel < = 55dB, then CR = 1.43:1 (or closest value)
- else if HearingThresholdLevel < = 60dB, then CR = 1.54:1 (or closest value)
- else if HearingThresholdLevel < = 65dB, then CR = 1.67:1 (or closest value)
- else if HearingThresholdLevel > 65dB, then CR = 1.82:1 (or closest value)
- Adjust overall Gain to match Soft Gain to Soft Target at 3kHz. If not OTE or RIC style instrument, then use formula to match 3kHz or appropriate peak.
- Equalizer
Match all Soft Gain frequencies to Soft Target, from 3kHz and below- Match all Soft Gain frequencies to Soft Target, from 3kHz and below
(except OTE with Tubing Type ⇒ OTE Thin Tube, or RIC with Vent Type ⇒ RIC Open)(NOTE: The OTE exception is no longer necessary since the introduction of REAR adjustments to avoid negative gain in low frequencies (following Steve Armstrong's algorithm)) — Siegwart Mayr 2009/03/13 10:50 Equalizer (except OTE with Tubing Type > OTE Thin Tube, or RIC with Vent Type > RIC Open) = Reduce 200Hz & 500Hz by 2dBEqualizer (for OTE with Tubing Type > OTE Thin Tube, or RIC with Vent Type > RIC Open) = Match Soft Gain at 200Hz & 500Hz to Software Target.Equalizer (except OTE with Tubing Type > BTE Ear Hook, or RIC with Vent Type > RIC Custom Mold) = Reduce 200Hz & 500Hz by 2dBEqualizer for OTE with Tubing Type > BTE Ear Hook, or RIC with Vent Type > RIC Custom Mold = Match Soft Gain at 200Hz & 500Hz to Software Target.- Equalizer = Reduce 200Hz & 500Hz by 2dB
- Equalizer for OTE with Tubing Type ⇒ OTE Thin Tube, or RIC with Vent Type ⇒ RIC Open = Reduce 1.0kHz, 4.2kHz, & 5.2kHz by 4dB.
- Match all Soft Gain frequencies to Soft Target from 3.5kHz to 4.2kHz
- Noise Reduction = On at minimum
- Calculate VC Range (if available)
- Perform Normal (WDRC) autofit
- Adaptive Directionality (AD) = Apply AD, first setting
- Calculate VC Range (if available)
- Perform Linear autofit
Custom Settings:All except DigitalOne4AFC (ClariD) and Spin: if Hearing Threshold Level at 1kHz < 30dB, set Noise Reduction at Low (8db), else set at Off (Default for Linear autofit)DigitalOne4AFC (ClariD): if Hearing Threshold Level at 1kHz < 30dB, set Expansion to 40dB, else set to Off (Default for Linear autofit)
- Set MPO (AGC-o) in all channels as follows:
- If Hearing Loss Threshold at 1kHz < 20dB, then MPO = -12dB or closest value
- If Hearing Loss Threshold at 1kHz > 20dB and < 50dB, then MPO = -8dB or closest value
- If Hearing Loss Threshold at 1kHz > = 50dB, then MPO = -4dB or closest value
- Show Soft and Loud Targets only
- Calculate VC Range (if available)
- Perform Normal (WDRC) autofit
- Compression Ratio = 1:1 (all channels)
- Noise Reduction = Off (if available)
- Show Soft Target only
- Calculate VC Range (if available)
- Perform Normal (WDRC) autofit.
- Prompt for Directional Mics (if Half Shell or Full Shell), or detect whether instrument has built-in Directional Mics.
- If answer is No to directional mics, or not a Half Shell or Full Shell, then:
- Compression Ratio = Increase by 1 step (next setting above) in first half of channels.
- Equalizer = Reduce 200Hz & 500Hz by 4dB.
- Gain = Reduce by 2dB.
- Noise Reduction = Medium setting.
- Else if answer is Yes to Directional Mics, then:
- Input = Cardioid/Directional.
- Noise Reduction = Medium setting.
- Custom Settings:
- Sparo, SparoAD, nVe4, nVeAD, Flx (all Open Fit perhaps?):
- Equalizer = Match all frequencies to Soft Target from 2.5 kHz - 4.2 kHz.
- Gain = Reduce by 2dB.
- Calculate VC Range (if available).
- Perform Linear autofit.
- Equalizer = Set 4.2kHz & above to minimum.
- Equalizer = Set 200Hz & 500Hz to minimum.
- Prompt for Telecoil, or detect it (do not prompt) when using:
- RIC or BTE style instruments. These have it built-in. Answer = Yes.
CIC or MiniCanal style instruments. These cannot support a Telecoil. Answer = No.
- If answer is Yes to Telecoil, then:
- Input = Telecoil.
- Else if answer is No to Telecoil, then:
- Input = Omni-directional Mic.
- Gain = Reduce by 3 dB.
- Equalizer = Reduce 3kHz by 4 dB (2 steps).
- Show Soft Target only.
- Calculate VC Range (if available).
- Perform Normal (WDRC) autofit
- Compression Ratio = 1.5:1 or closest value (all channels)
- Gain = Reduce by 4 dB
- Noise Reduction = Off (if available)
- Adaptive Feedback Canceler = Off (if available)
- Equalizer = Increase 200Hz, 500Hz, & 1kHz by 2 dB
- Calculate VC Range (if available)
- Perform Normal (WDRC) autofit.
- Noise Reduction = Off (if available).
- Equalizer = Increase 500Hz & 1kHz by 2dB.
- Compression Ratio = Reduce all channels by 1 step.
- Calculate VC Range (if available).
- Detect whether using directional mics (read code in scratch memory). (Currently prompting for directional mics but should not) — Jenny Sudduth 2008/09/10 18:32
- If instrument has built-in directional mics (Sparo, SparoAD, InTune (except Intuition4+), Ethos), then:
- Set Input = Cardioid by performing Restaurant/Party autofit
- Bass boost = On (if available) (nVeAD, not turning On bass boost currently) — Jenny Sudduth 2008/09/10 18:57
- Else if no built-in directional mics (Spin, Sparo2, DigitalOne4NRPlus, Intuition4+, Ethos), then:
- Set Input = Omni Mic by performing Television autofit
- Bass boost = Off (if available)
- Noise Reduction = Low setting (if available, else Expansion TK = 40dB)
- Custom Settings:
Sparo, SparoAD:Noise Reduction = Low setting (if available)(redundant with Restaurant/Party autofit)Input = Cardioid(redundant with Restaurant/Party autofit)
Intuition4+, Ethos: Bass boost = Off (if available)InTune (except Intuition4+): Bass boost = On (if available)- All except Intuition4+:
- Equalizer: Increase 500 Hz & 1 kHz by 2 dB.
- Compression: Reduce Compression Ratio in Ch. 2 & 3 by 2 steps (Ethos: Ch. 3 - 6).
- Calculate VC Range (if available)
- Perform Normal (WDRC) autofit
- Prompt for Directional Mics (if Half Shell or Full Shell), or detect if available built-in (Sparo, Sparo AD, Sparo 8, Intuition 4AD, nVeAD, Flx)
- If answer is Yes to Directional Mics, then:
- Gain = Reduce by 2 dB
- Input = Hypercardioid/Directional
- Noise Reduction = Highest setting
- Else if answer is No to Directional Mics, then:
- Equalizer = Reduce 200Hz & 500Hz by 4dB.
- Gain = Reduce by 4 dB
- Compression Ratio = Increase first half of channels by 3 steps
- Compression Ratio = Increase last half of channels by 2 steps
- MPO (AGC-o) = Midpoint (all channels)
- Noise Reduction = High (if available)
- Custom Settings:
- Sparo, SparoAD, nVe4, nVeAD, Flx:
- Equalizer = Match all frequencies to Soft Target from 2 kHz - 4.2 kHz
- Reduce overall Gain by 4 dB
- Calculate VC Range (if available)
Abbreviation | Term |
---|---|
AGC-o | Automatic Gain Control (output) |
Avg | Average |
BTE | Behind-the-Ear instrument |
Ch | Channel |
CR | Compression Ratio |
dB | Decibels |
Freq | Frequency |
HL | Hearing Level (or Loss) as depicted in an Audiogram (aka HTL) |
HTL | Hearing Threshold Level as depicted in an Audiogram (aka HL) |
Hz | Hertz |
kHz | KiloHertz |
MPO | Maximum Power Output |
OTE | Other-the-Ear instrument |
RIC | Receiver-in-Canal instrument |
SRT | Speech Reception Threshold |
TK | Threshold Kneepoint |
VC | Volume Control |
- WDRC Autofit for Accurio (rev 2006-08-01)